File to Read File Again C++
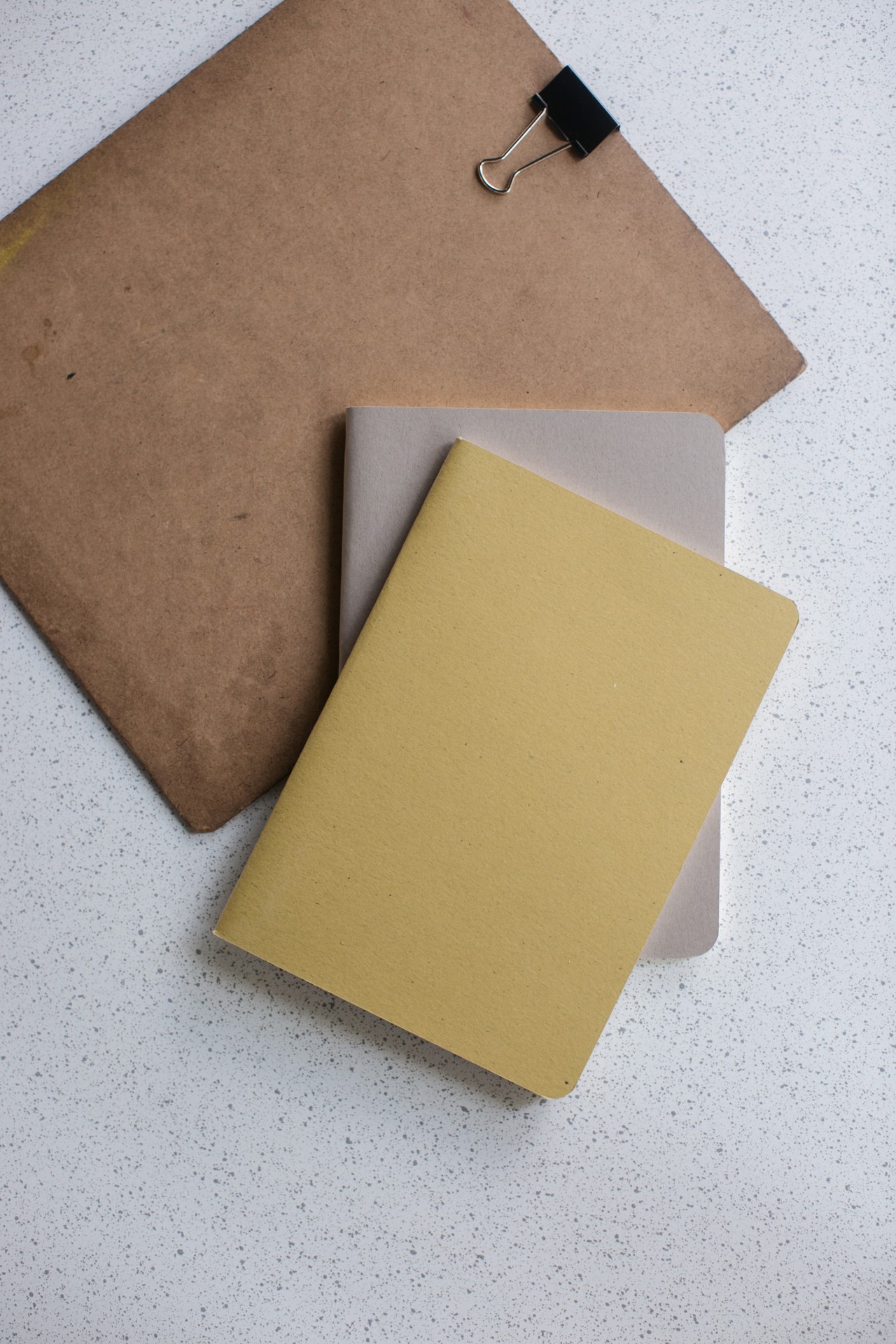
If you've written the C helloworld
programme before, you already know basic file I/O in C:
/* A elementary hello earth in C. */ #include <stdlib.h> // Import IO functions. #include <stdio.h> int main() { // This printf is where all the file IO magic happens! // How heady! printf("Hello, world!\n"); return EXIT_SUCCESS; }
File handling is one of the almost important parts of programming. In C, we utilize a structure pointer of a file type to declare a file:
FILE *fp;
C provides a number of build-in function to perform bones file operations:
-
fopen()
- create a new file or open a existing file -
fclose()
- shut a file -
getc()
- reads a character from a file -
putc()
- writes a graphic symbol to a file -
fscanf()
- reads a set of data from a file -
fprintf()
- writes a set of data to a file -
getw()
- reads a integer from a file -
putw()
- writes a integer to a file -
fseek()
- set the position to want point -
ftell()
- gives electric current position in the file -
rewind()
- set the position to the beginning point
Opening a file
The fopen()
part is used to create a file or open an existing file:
fp = fopen(const char filename,const char style);
There are many modes for opening a file:
-
r
- open a file in read style -
w
- opens or create a text file in write mode -
a
- opens a file in append manner -
r+
- opens a file in both read and write mode -
a+
- opens a file in both read and write mode -
west+
- opens a file in both read and write mode
Here'due south an example of reading information from a file and writing to it:
#include<stdio.h> #include<conio.h> main() { FILE *fp; char ch; fp = fopen("hullo.txt", "w"); printf("Enter data"); while( (ch = getchar()) != EOF) { putc(ch,fp); } fclose(fp); fp = fopen("hello.txt", "r"); while( (ch = getc(fp)! = EOF) printf("%c",ch); fclose(fp); }
Now you lot might exist thinking, "This simply prints text to the screen. How is this file IO?"
The reply isn't obvious at first, and needs some agreement virtually the UNIX system. In a UNIX system, everything is treated as a file, meaning yous can read from and write to it.
This means that your printer can be bathetic equally a file since all you do with a printer is write with information technology. Information technology is as well useful to call up of these files every bit streams, since as you'll see later, you can redirect them with the trounce.
So how does this relate to helloworld
and file IO?
When you telephone call printf
, you are really but writing to a special file called stdout
, short for standard output . stdout
represents the standard output equally decided by your vanquish, which is usually the last. This explains why it printed to your screen.
There are two other streams (i.east. files) that are available to y'all with endeavor, stdin
and stderr
. stdin
represents the standard input , which your shell usually attaches to the keyboard. stderr
represents the standard error output, which your vanquish usually attaches to the terminal.
Rudimentary File IO, or How I Learned to Lay Pipes
Enough theory, let's go downwardly to concern by writing some code! The easiest manner to write to a file is to redirect the output stream using the output redirect tool, >
.
If you desire to append, you can apply >>
:
# This volition output to the screen... ./helloworld # ...but this will write to a file! ./helloworld > hullo.txt
The contents of hello.txt
will, not surprisingly, be
Hello, world!
Say we have some other program called greet
, like to helloworld
, that greets you with a given name
:
#include <stdio.h> #include <stdlib.h> int main() { // Initialize an array to hold the name. char proper noun[twenty]; // Read a string and save it to name. scanf("%due south", name); // Print the greeting. printf("Hello, %s!", name); return EXIT_SUCCESS; }
Instead of reading from the keyboard, nosotros can redirect stdin
to read from a file using the <
tool:
# Write a file containing a name. echo Kamala > name.txt # This volition read the name from the file and print out the greeting to the screen. ./greet < name.txt # ==> Hello, Kamala! # If you wanted to also write the greeting to a file, you could do so using ">".
Note: these redirection operators are in bash
and similar shells.
The Real Deal
The in a higher place methods only worked for the most basic of cases. If you wanted to do bigger and better things, you will probably desire to work with files from within C instead of through the shell.
To accomplish this, y'all will use a role called fopen
. This function takes 2 cord parameters, the first being the file name and the second being the mode.
The mode are basically permissions, so r
for read, w
for write, a
for append. You tin as well combine them, so rw
would mean you lot could read and write to the file. At that place are more modes, only these are the most usually used.
Later on you have a FILE
pointer, you lot can utilize basically the same IO commands you would've used, except that you take to prefix them with f
and the kickoff argument volition be the file arrow. For example, printf
's file version is fprintf
.
Here's a program called greetings
that reads a from a file containing a list of names and write the greetings to another file:
#include <stdio.h> #include <stdlib.h> int primary() { // Create file pointers. FILE *names = fopen("names.txt", "r"); FILE *greet = fopen("greet.txt", "w"); // Check that everything is OK. if (!names || !greet) { fprintf(stderr, "File opening failed!\n"); return EXIT_FAILURE; } // Greetings time! char proper noun[twenty]; // Basically proceed on reading untill at that place'due south nothing left. while (fscanf(names, "%due south\n", proper name) > 0) { fprintf(greet, "Hello, %southward!\due north", name); } // When reached the end, impress a message to the concluding to inform the user. if (feof(names)) { printf("Greetings are washed!\n"); } render EXIT_SUCCESS; }
Suppose names.txt
contains the post-obit:
Kamala Logan Carol
So after running greetings
the file greet.txt
will contain:
Hi, Kamala! Hello, Logan! Hullo, Ballad!
Acquire to code for free. freeCodeCamp's open up source curriculum has helped more 40,000 people get jobs as developers. Get started
Source: https://www.freecodecamp.org/news/file-handling-in-c-how-to-open-close-and-write-to-files/
0 Response to "File to Read File Again C++"
Publicar un comentario